Sample Codes
The best method to understand
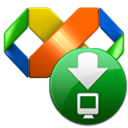
Demo Project VB.Net
Make Sure that Tally Server is running before running this sample project.
(Minimize tally with selected company to which vouchers are to be imported)
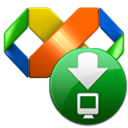
Demo Project C#
Make Sure that Tally Server is running before running this sample project.
(Minimize tally with selected company to which vouchers are to be imported)
Introduction
Tally Dll is a Dynamic Link Library (DLL) that allows Software developers to Integrate their Applications with Tally Accounting Software. Using tallydll, developers can push or pull data from Tally Software in real-time.
It is based on the Client/Server principles which are as follows:-
The Client Application sends a Request to the Tally Server. The request may be either to Write data (Import data into Tally) or Read data (Export data from Tally).
The Server processes the request and sends a response back to the client.
Demo Project
Please Click Here to download full Demo Project explaining how to Post Purchase, Sales, Payment, Receipts and how to create masters like Ledger, Stock Item, Units etc.
Functions
CREATELEDGER(objLedger)
This function creates a ledger in tally with out any xml tags.
objLedger is a collection of fields for the creation of Account Ledger, configure and set the object's properties
Usage
Dim oDll as TallyDll.tallydll = New TallyDll.tallydll
Dim objLedger as Ledger = New Ledger
objLedger.LEDGERNAME="XYZ"
objLedger.PARENT="Sundry Debtors"
objLedger.OPENINGBALANCE=-100
oDll.CREATELEDGER(objLedger)
TallyDll.tallydll oDll = new TallyDll.tallydll();
Ledger objLedger = new Ledger();
objLedger.LEDGERNAME = "XYZ";
objLedger.PARENT = "Sundry Debtors";
objLedger.OPENINGBALANCE = -100;
oDll.CREATELEDGER(objLedger);
CREATELEDGER_Async(objLedger)
This function creates a ledger in tally with out any xml tags.
objLedger is a collection of fields for the creation of Account Ledger, configure and set the object's properties
Usage
Dim oDll as TallyDll.tallydll = New TallyDll.tallydll
Dim objLedger as Ledger = New Ledger
objLedger.LEDGERNAME="XYZ"
objLedger.PARENT="Sundry Debtors"
objLedger.OPENINGBALANCE=-100
oDll.CREATELEDGER_Async(objLedger)
TallyDll.tallydll oDll = new TallyDll.tallydll();
Ledger objLedger = new Ledger();
objLedger.LEDGERNAME = "XYZ";
objLedger.PARENT = "Sundry Debtors";
objLedger.OPENINGBALANCE = -100;
oDll.CREATELEDGER_Async(objLedger);
CREATELEDGERGROUP(objLedgerGroup)
This function creates a ledger group in tally with out any xml tags.
objLedgerGroup is a collection of fields for the creation of Ledger Group under parent group, configure and set the object's properties
Usage
Dim oDll as TallyDll.tallydll = New TallyDll.tallydll
Dim objLedgerGroup as Ledger = New LedgerGroup
objLedger.GROUPNAME="My Group"
objLedger.PARENT="Sundry Creditors"
oDll.CREATELEDGERGROUP(objLedgerGroup)
TallyDll.tallydll oDll = new TallyDll.tallydll();
LedgerGroup objLedgerGroup = new LedgerGroup();
objLedger.GROUPNAME = "My Group";
objLedger.PARENT = "Sundry Creditors";
oDll.CREATELEDGERGROUP(objLedgerGroup);
REQUESTENTRY(xml)
xml- is the xml string for requesting data from or to tally server.
Click here for the list of xml formats that used to send to the tally server
This function returns an xmlDocument which has the status of requested parameter
<ENVELOPE>
<HEADER>
<TALLYREQUEST>Import Data</TALLYREQUEST>
</HEADER>
<BODY>
<IMPORTDATA>
<REQUESTDESC>
<REPORTNAME>All Masters</REPORTNAME>
</REQUESTDESC>
<REQUESTDATA>
<TALLYMESSAGE xmlns:UDF="TallyUDF">
<GROUP NAME="My Debtors" ACTION="Create">
<NAME.LIST>
<NAME>My Debtors</NAME>
</NAME.LIST>
<PARENT>Sundry Debtors</PARENT>
<ISSUBLEDGER>No</ISSUBLEDGER>
<ISBILLWISEON>No</ISBILLWISEON>
<ISCOSTCENTRESON>No</ISCOSTCENTRESON>
</GROUP>
</TALLYMESSAGE>
</REQUESTDATA>
</IMPORTDATA>
</BODY>
</ENVELOPE>
Tags: Tally Integration, C# to Tally, VB.NET to Tally